PROJECT: FreeTime
1. Overview
FreeTime is a desktop application that helps users coordinate their schedules with their friends or groupmates. It allows users to add and remove events from their timetables. It also uses these individual user timetables to generate a combined timetable showing when everyone the user wants to meet is available.
FreeTime receives input through a command line interface (CLI) while displaying output through a graphical user interface (GUI). It is written in Java, and has approximately 10 kLoC.
FreeTime was developed in a team of four throughout the course of a semester. It is modified from the Address Book (Level 4) (AB4) project by SE-EDU.
FreeTime was developed as part of the module requirements for the following modules offered by the School of Computing, National University of Singapore:
-
CS2113 - Software Engineering & Object-Oriented Programming
-
CS2101 - Effective Communication for Computing Professionals
This project portfolio documents my contributions to the FreeTime project.
2. Summary of contributions
-
Major enhancement: Added timetables to FreeTime
-
What it does: Implements timetables for each user and allows the user to add and remove timeslots from their own timetables. Additionally, allows users to determine when they can organise meetings such that everyone they want to meet can be present.
-
Justification: As it is difficult for students with varied and complex timetables to schedule meetings for their many group projects, FreeTime simplifies this process by automatically highlighting mutually free timeslots among different user timetables.
-
Highlights: This enhancement was challenging as it required the development of a completely new portion of the GUI for the displaying of timetables, which required proficiency in JavaFX, the software platform used to build FreeTime’s GUI. Moreover, significant additions were made to the Model component to allow the in-app storage of timetables.
-
-
Other contributions:
-
Project management:
-
Managed releases
v1.1
-v1.4
on GitHub (4 releases)
-
-
Community
-
Tools:
-
Miscellaneous enhancements to new and existing features:
-
3. Contributions to the User Guide
This section details my contributions to FreeTime’s User Guide.
3.1. Add a timeslot to your timetable: add
(a
)
Adds a timeslot to your timetable.
Format: add Monday 10:00-12:30
Examples:
-
add Monday 10:00-12:30
Adds the timeslot from 10:00 to 12:30 on Monday to your timetable. -
add Fri 13:30-14:00
Adds the timeslot from 13:30 to 14:00 on Friday to your timetable. -
add Wed 17-18
Adds the timeslot from 17:00 to 18:00 on Wednesday to your timetable.
After adding a timeslot, you should see the following:
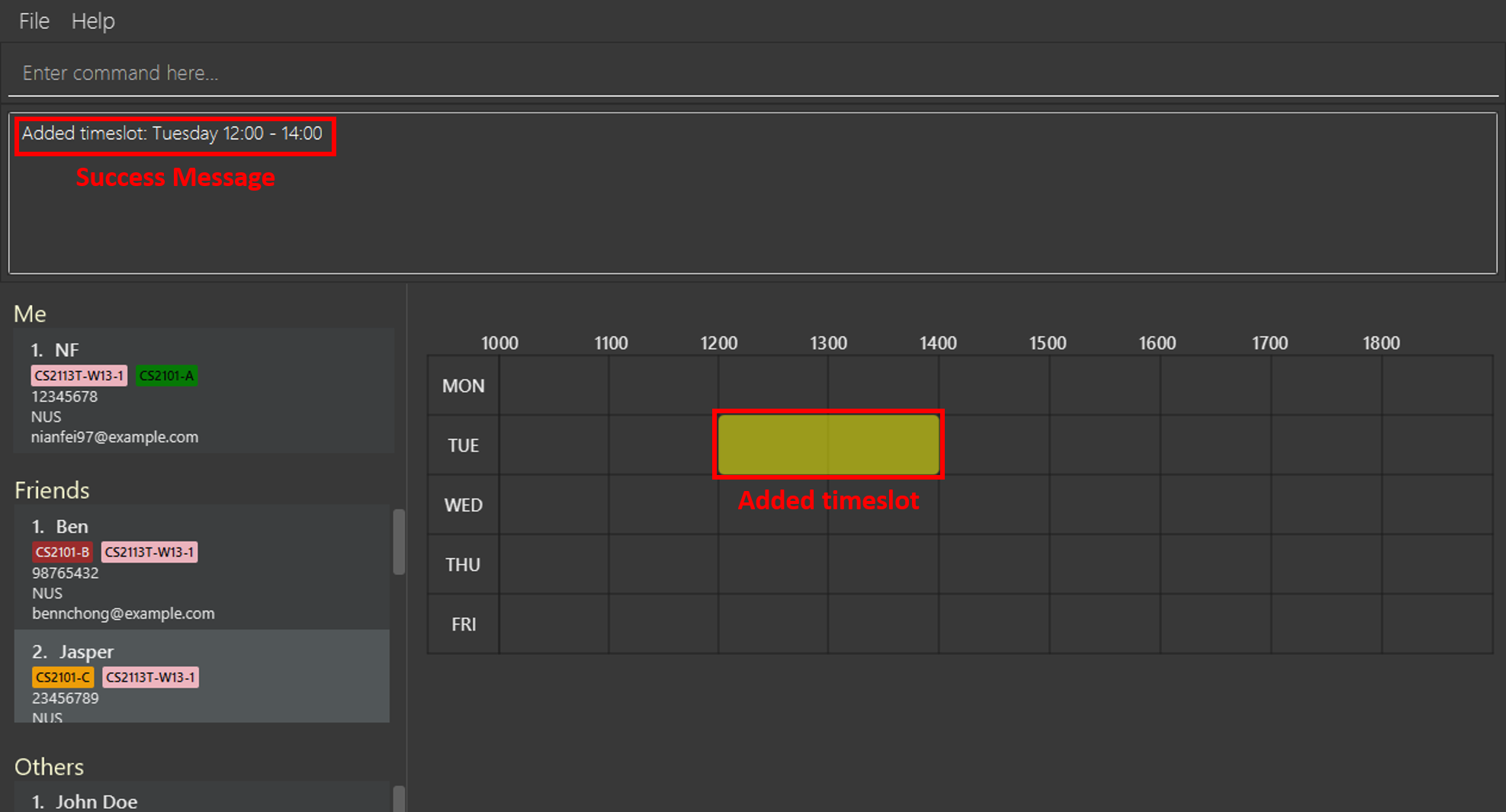
3.2. Delete a timeslot from your timetable: delete
(d
)
Deletes a timeslot from your timetable.
Format: delete Monday 10:00-12:30
Examples:
-
delete Monday 10:00-12:30
Deletes the timeslot from 10:00 to 12:00 on Monday from your timetable. -
delete Fri 13:30-14:00
Deletes the timeslot from 13:30 to 14:00 on Friday from your timetable. -
delete Wed 17-18
Deletes the timeslot from 17:00 to 18:00 on Wednesday from your timetable.
3.3. Show free slots among selected people: free
(fr
)
Highlights timeslots where you and everyone specified is free.
Format: free INDEX…
Examples:
-
free 1 2
Highlights timeslots where you, friend 1, and friend 2, are all free to meet up.
After executing the command, you should see the following:
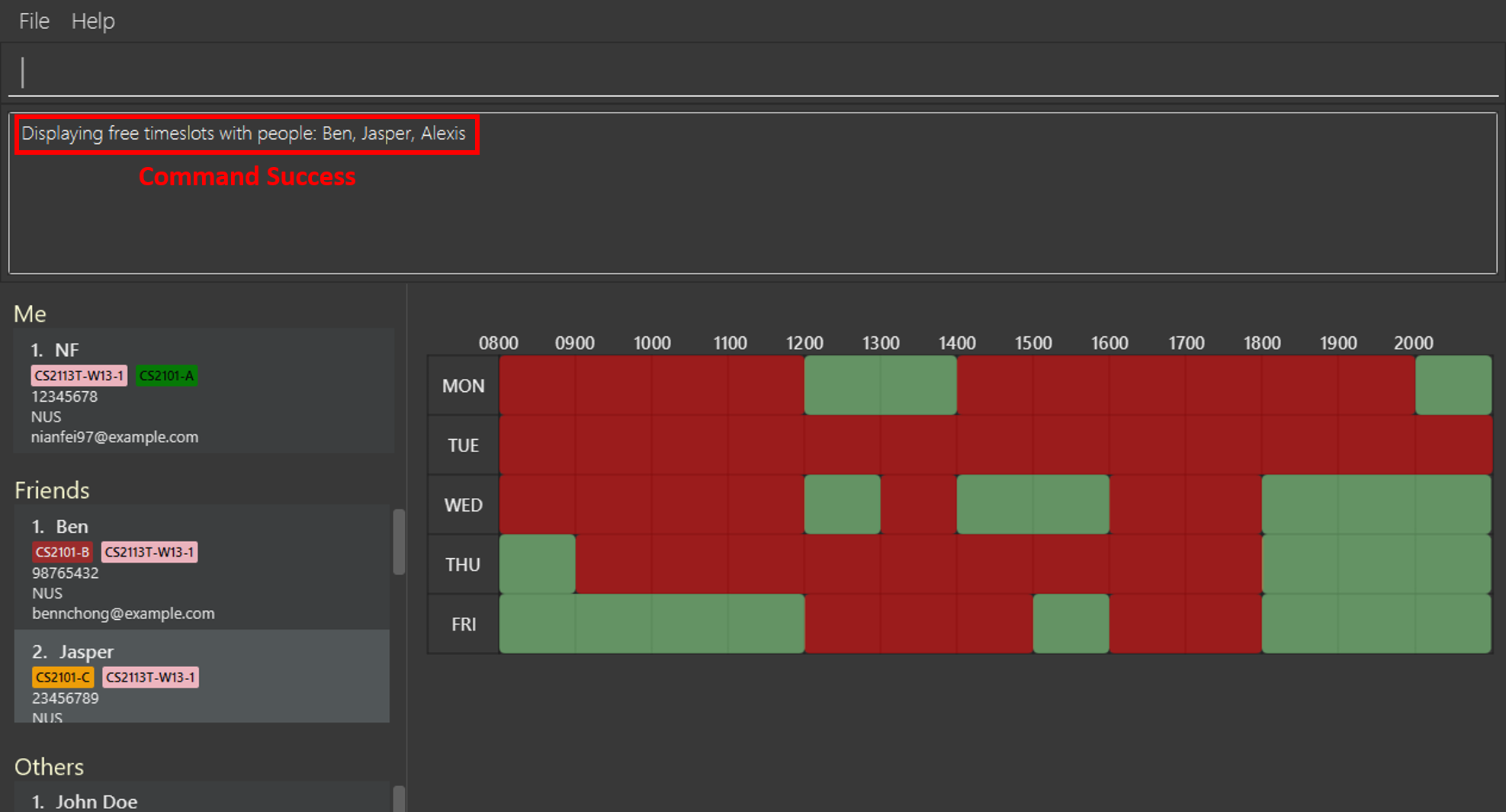
4. Contributions to the Developer Guide
This section details my contributions to FreeTime’s Developer Guide.
4.1. Timetable feature
4.1.1. Current implementation
FreeTime’s timetable feature allows users to store and view their own timetables.
FreeTime can also display a deconflicted timetable, highlighting mutually free timeslots among the current user and all other selected people.
The timetable feature can be broadly split into two parts:
1. The backend, which handles the storage and logic of TimeTable
objects;
2. The frontend, which handles the display of TimeTable
objects.
Backend implementation
The TimeTable
object is composed under the Person
class in Model
. Each TimeTable
is composed of any number of TimeSlot
objects. Each TimeSlot
consists of:
1. One DayOfWeek
object to indicate the day of week of the TimeSlot
;
2. Two LocalTime
objects to indicate the start time and end time of the TimeSlot
respectively.
3. One Color
object to indicate the color of the TimeSlot
when displayed on FreeTime’s UI.
The following class diagram summarises the relationship between the components of the TimeTable
class:
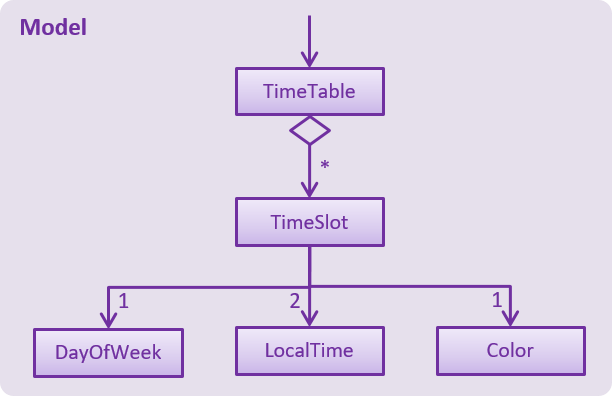
TimeTable
ClassThe TimeTable
class implements two key methods:
1. TimeTable#addTimeSlot()
- to add a new TimeSlot
to the TimeTable
2. TimeTable#deleteTimeSlot()
- to remove an existing TimeSlot
from the TimeTable
The class DeconflictTimeTable
, which inherits from TimeTable
, is used when the free
command is executed to store mutually free timeslots among users.
When the free
command is executed, a DeconflictTimeTable
object is instantiated with all TimeSlot
objects in the user’s TimeTable
. Subsequently, the TimeTable
objects of every Person
that is passed as an argument to the free
command is added to the DeconflictTimeTable
.
The following sequence diagram shows the significant method calls for the method FreeCommand#execute()
:
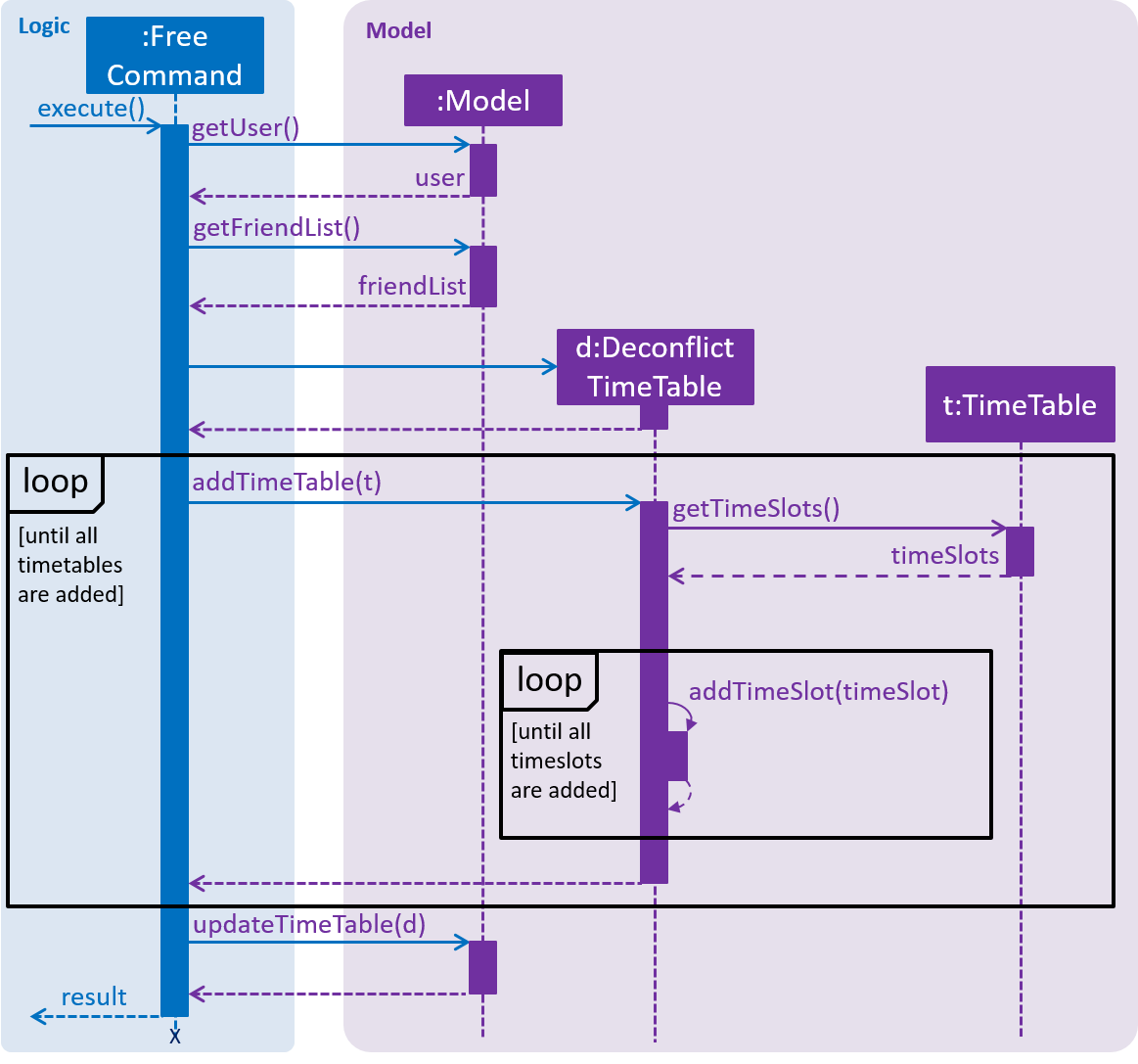
FreeTime#execute()
MethodThe key difference between TimeTable
and DeconflictTimeTable
lies in the implementation of the addTimeSlot()
method. TimeTable#addTimeSlot()
throws a TimeSlotOverlapException
when the TimeSlot
to be added overlaps with an existing TimeSlot
in the TimeTable
.
However, DeconflictTimeTable#addTimeSlot()
merges the TimeSlot
to be added with all overlapping TimeSlot
objects in the DeconflictTimeTable
. Thus, DeconflictTimeTable#addTimeSlot()
never throws TimeSlotOverlapException
.
Frontend implementation
TimeTablePanel
extends the abstract class UIPart
and is implemented using a BorderPane
. It is composed of the following classes:
1. TimeTableDayMarkerGrid
- a GridPane
on the left of the TimeTablePanel
to display the days of the week;
2. TimeTableTimeMarkerGrid
- a GridPane
on the top of the TimeTablePanel
to display the time markers
3. TimeTableMainGrid
- a GridPane
in the center of the TimeTablePanel
. Composed of any number of TimeTablePanelTimeSlot
objects.
The following class diagram summarises the relationship between the components of the TimeTablePanel class:
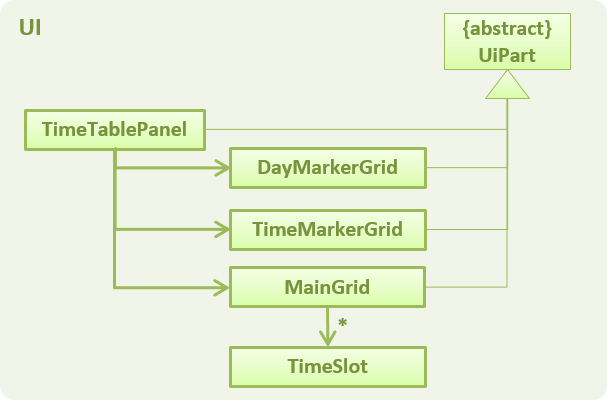
TimeTablePanel
ClassThe following screenshot shows the relative position of all the components in TimeTablePanel
:
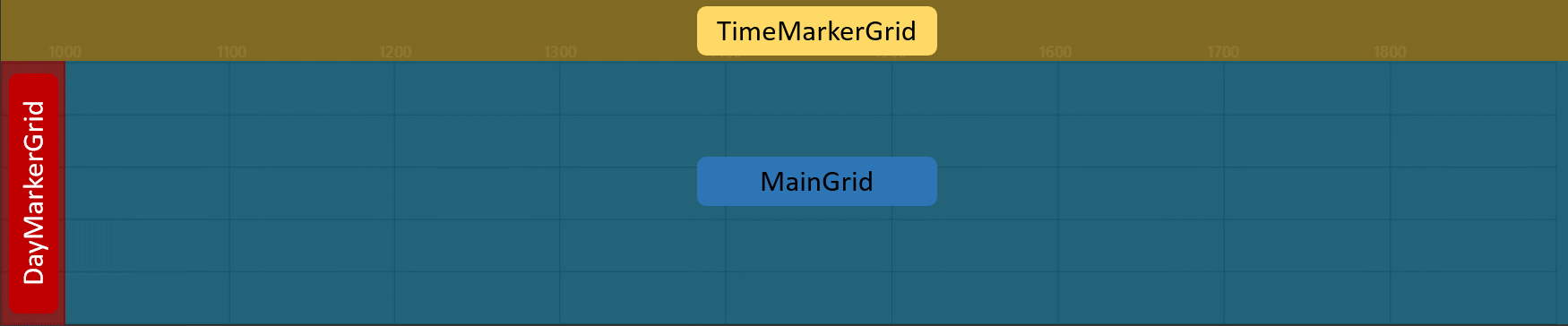
FreeTime’s UI is updated through the EventsCenter
every time Model#updateTimeTable()
is called.
The following sequence diagram shows the significant method calls for the method Model#updateTimeTable()
.
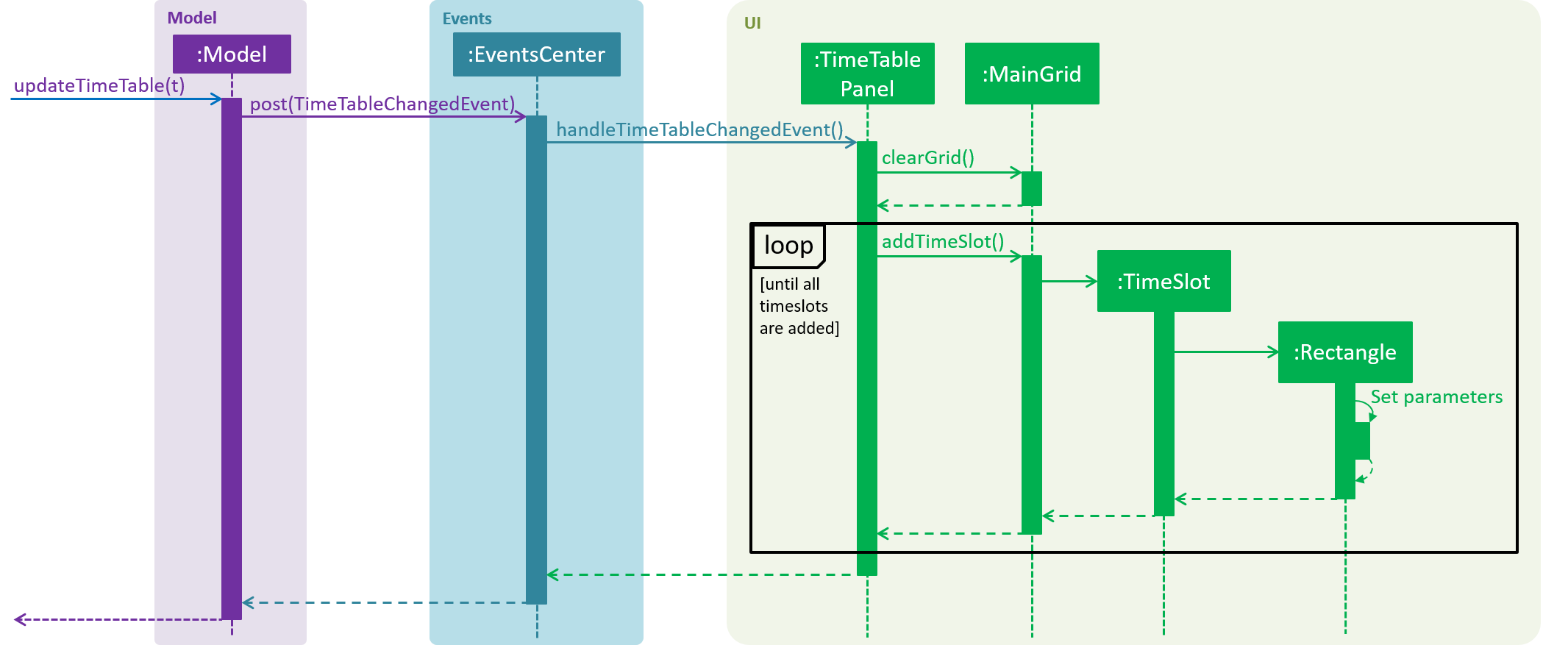
FreeTime#execute()
Method
4.1.2. Design Considerations
Aspect: How the frontend updates the TimeTable
to be displayed
-
Alternative 1 (current choice): Clears the entire
TimeTableMainGrid
before loading the newTimeTable
-
Pros: Easy to implement, only one method (
loadTimeTable()
) is required -
Cons: May suffer from performance degradation, especially when the
TimeTable
to be loaded contains manyTimeSlot
objects
-
-
Alternative 2: Detect the difference between the currently displayed
TimeTable
before adding or deletingTimeTablePanelTimeSlot
objects accordingly.-
Pros: Reduces execution time of methods which update the displayed
TimeTable
-
Cons: A method to detect the difference between
TimeTable
objects must be implemented. May not result in significant performance improvements when switching betweenTimeTable
objects with few or noTimeSlot
objects in common.
-