Overview
FreeTime is a desktop application targeted at students who have many projects that require meeting many people at different times. FreeTime aims to reduce the hassle of find available timeslots in which everyone is mutually available. This project is part of the deliverables for the following modules offered by the School of Computing in the National University of Singapore:
-
CS2113T - Software Engineering and Object-Oriented Programming
-
CS2101 - Communication for Computing Professionals
The user interacts with it using a Command Line Interface (CLI), and it has a Graphical User Interface (GUI) created with JavaFX. It is written in Java, and has about 10 kLoC.
This project portfolio shows my contributions to the project, which includes the learning the Java Programing Language and JavaFX.
Summary of contributions
-
Major enhancement: added the concept of friends and the ability to friend/unfriend a user in the database
-
What it does: Invoking the
friend
command will add the selected person to your friend list.unfriend
will do the remove an existing friend from the friend list. -
Justification: The idea of the application is to work as a social platform where users can look for other users with similar modules and to coordinate timetables with them. Before adding a particular user as a friend, the timetables and other personal information about the user will be obscured. This is to address privacy concerns of users revealing too much information to unknown people.
-
Highlights: Implementing this concept and commands required that the main UI to be modified and changes how the logic works fundamentally. I had to learn how to use JavaFX to manage the different panels to show the friends and users individually. The implementation too was challenging as it required changes to the main logic of the code and many tests were changed as a result.
-
-
Minor enhancement: added a tag command that allows the user to search for persons by module code. This is to enhance the search feature in the case where there are too many users in the database.
-
Other contributions:
-
Enhancements to existing features:
-
Designed and updated the existing application icon and title.
-
Added colors to group tags
-
-
Documentation:
-
Ensured consistency in the UserGuide.
-
-
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Locating persons by group : group
(g
)
Find persons whose groups match the specified groups.
Format: group GROUPNAME [MORE_GROUPNAMES]
Example:
-
group CS2101
Filters both the others list and the friends list to show only users with the group "CS2101". -
group CS2101 CS2113T
Filters both the others list and the friends list to show only users with both group "CS2101" and "CS2113T".
Use the command listall to revert the panels back to its original state.
|
Adding a person to your friend list : friend
(af
)
Adds a person from the others list to your friend list.
Format: friend INDEX
|
Examples:
-
friend 1
Adds the first user in the others list to your list of friends.-
Before executing the command, your window might look like the following, without friends:
Figure 1. Before adding friend to your friend list. -
After executing
friend 6
, there will be a success message and the first person should be added to the friend’s list, as follows:Figure 2. After adding friend to your friend list. -
More information about the user is now shown and commands such as
free
andselect
can now be used.
-
Removing a person from your friend list : unfriend
(uf
)
Removes a person from your friend list.
Format: unfriend INDEX
Example:
-
unfriend 1
Removes the first user from the friends list.-
Before executing the command, look for the index of the person you would like to remove from your list:
Figure 3. Before removing friend from friend list. -
After executing
unfriend 1
, there will be a success message and the first person should be removed from the friend’s list, as follows:Figure 4. After adding friend to your friend list. -
More information about the user is now shown and commands such as
free
can now be used.
-
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Friend feature
Feature description
The friend feature allows users to befriend and add other users to their friend list. Timetables and personal information is only viewable for users in your friend list and timetables can only be deconflicted within your friends.
Current implementation
The feature is implemented with two main parts. The frontend and the backend, which will be explained below.
Frontend implementation
On the left side users will now see two panes, one above for friends and one below for the rest of the users not inside the friend list.
PersonListPanel is adapted to now have two Stackpane
instead of one and is nested inside a Splitpane
in the VBox
.
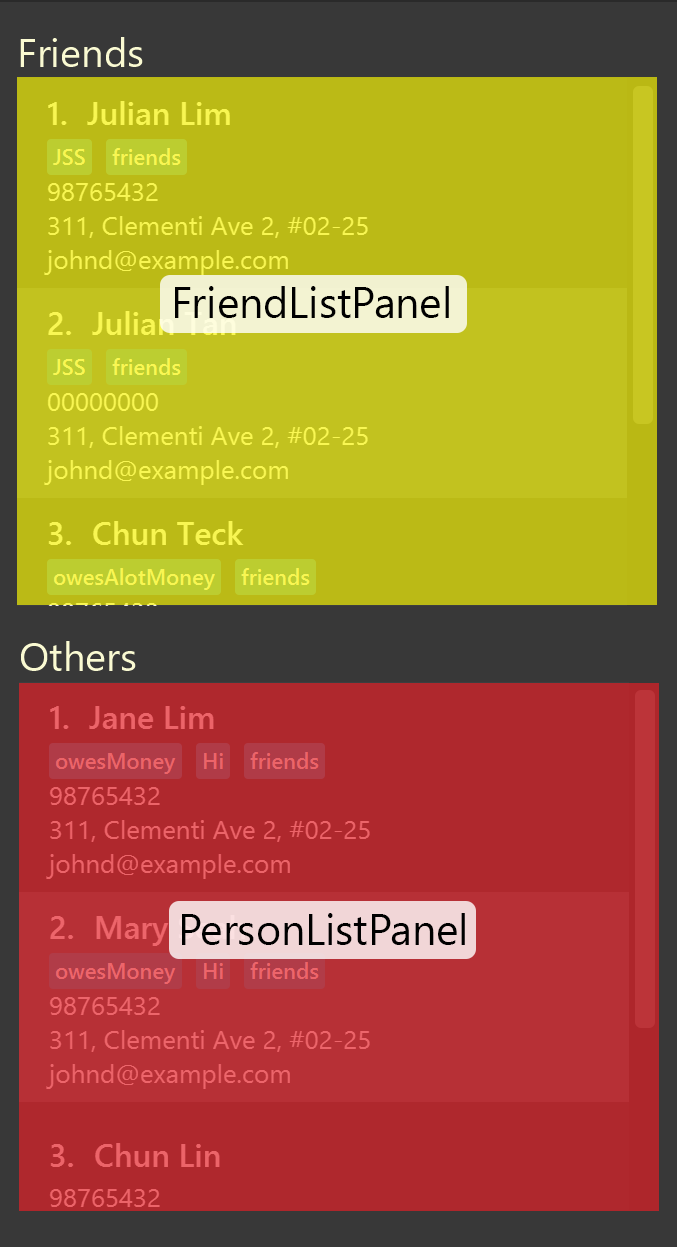
The two panels will be filtered based on two predicates, FriendListPredicate
and OtherListPredicate
.
The friends of the current user is iterated through and those that belong to the friend will be shown on the FriendListPanel.
The rest of the users will be shown on PersonListPanel. Both predicates will filter out the current user.
When additional filtering commands such as group
or find
are executed, the predicate produce from either of the commands will be added to a new CombinedFriendListPredicate
and CombinedOtherListPredicate
.
This creates a new predicate in which will filter the two panels accordingly.
Backend implementation
The User
is associated with a specific Person
. Each person can have any number of Friend
objects.
Every friend will have a Name
object that corresponds to the specific Person
object in the list.
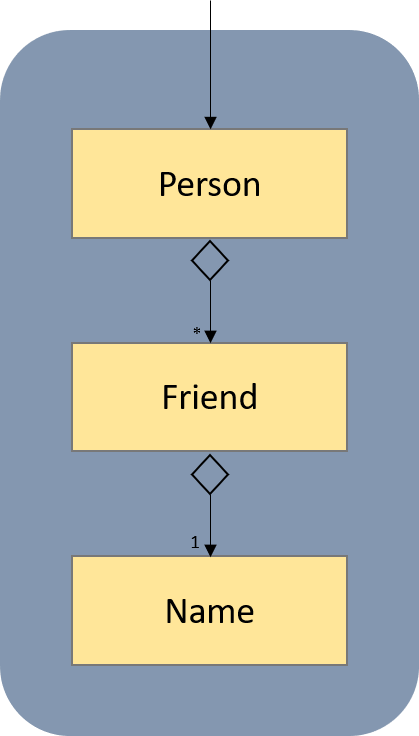
When the friend command is executed, the Person selected at the index will be added as a Friend
to the current user.
Likewise, the reverse will occur and the user will be added to the Person selected as a Friend
.
When the selected person logs in, he or she will see the user as a friend as well.
The following sequence diagram shows the execution of the Friend command:
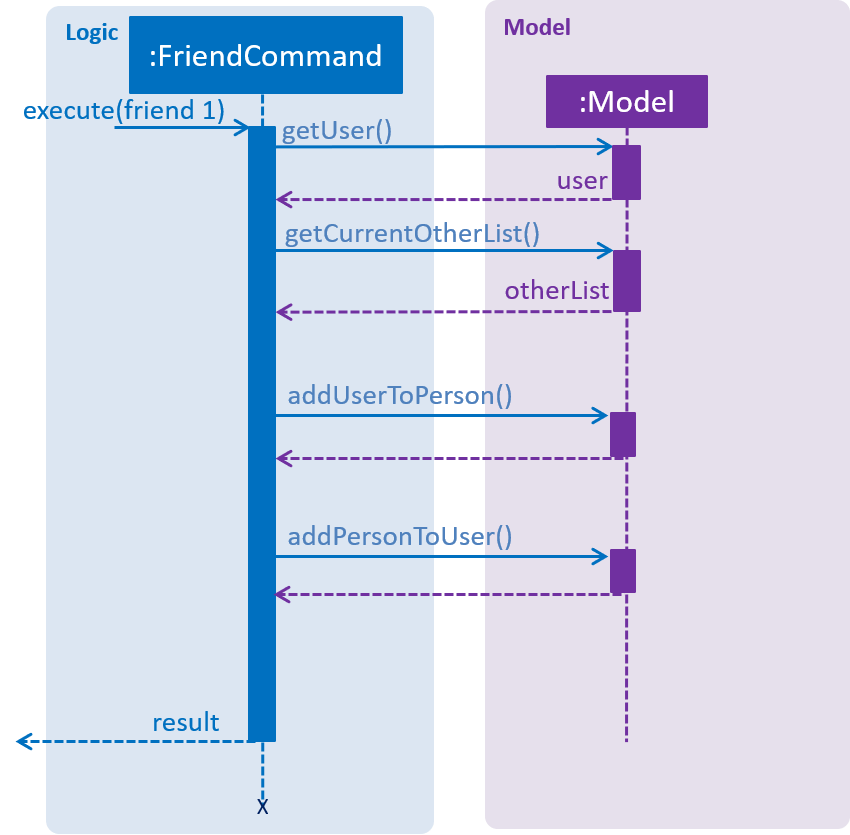
How to store the friends of the User
-
Alternative 1 (current choice): Every Friend of the user has a name which matches a specific person in the database
-
Pros: Every friend only includes essential information about the specific person
-
Cons: Takes longer to match the person in the friends list to a person in the database
-
-
Alternative 2: Allowing every Friend to compose a Person object
-
Pros: Friend contains more information that can be used
-
Cons: Duplicated entries of the same Person
-
How to display the friends and others of the current User
-
Alternative 1 (current choice): Use of predicates to set the list to reflect only the desired people.
-
Pros: Allow different predicates to be nested when more filtering commands are called. More dynamic filtering can be done.
-
Cons: The list internally contains all the users of the database, extra information is being loaded.
-
-
Alternative 2: Send a list of all friends and non-friends at the start of the application to individual panels.
-
Pros: Correct users will be loaded at the start for each panel.
-
Cons: Friends panel and others panel have to be repopulated again after every friend/unfriend
-